Laravel Sanctum: Simple Authentication For Your SPA
Last Updated on June 8, 2022
What is Laravel Sanctum?
Laravel Sanctum is a simple way for authenticating Single Page Applications(SPAs), Mobile Applications, and simple token-based APIs. It allows users to create multiple API tokens which they can use to access your application. The token themselves can have scopes that can be used to limit what can and cannot be accessed using the generated token.
Laravel provides multiple ways of authentication such as Social logins using Laravel Socialite, API authentication through Oauth using Laravel Passport, the basic session-based authentication using email and password and now API authentication without Oauth capabilities using Laravel Sanctum.
In this article, I am going to show you how to implement API authentication using Laravel Sanctum in your application.
How does Laravel Sanctum Work?
Laravel Sanctum is a simple package that is used to issue API tokens to users without the complication of OAuth. These tokens have a long expiration time but can be revoked at any time manually.
These Tokens are then stored in a single database table and can be authenticated by having them attached to the Authorization header.
This allows us to issue tokens from our server directly to our client/frontend applications.
Installation and Setup
To install the package, we can use the following command
composer require laravel/sanctum
The next step is to publish the Sanctum configurations and the migration files using the vendor:publish command.
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
We can then run the database migrations. These migrations create a table that stores the API tokens that are created.
php artisan migrate
Laravel Sanctum SPA Authentication
Once the configurations are done, we can now start authentication. The first step is to issue API tokens. These tokens are used to authenticate API requests to the application. The token must be included in the Authorization Header as a Bearer Token. The header should be presented as follows:
HEADERS {
'Content-Type': 'application/json',
'Authorization': 'Bearer <Token>'
}
In this case, the <Token> is the generated API token that is used to identify the user/client making the request.
To issue tokens for users, we first need to use the Laravel\Sanctum\HasApiTokens trait in our User model.
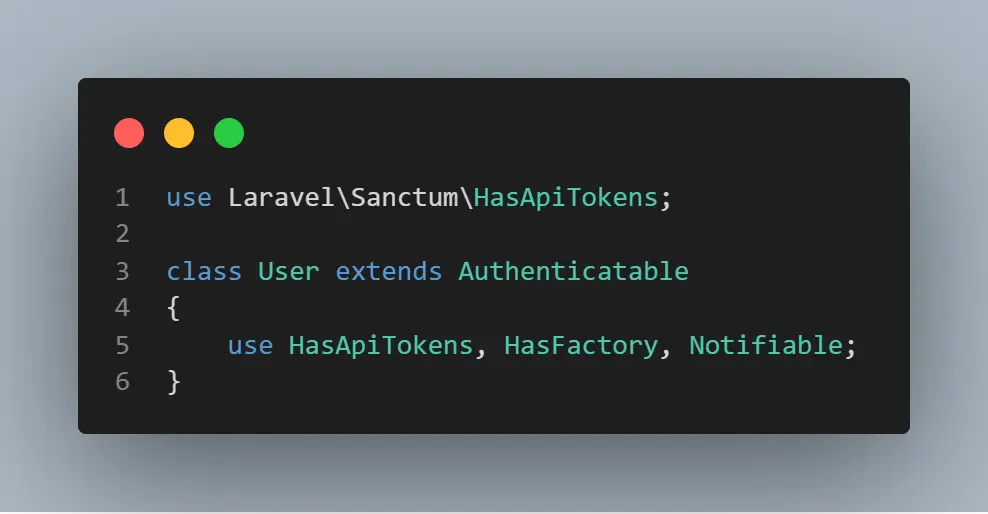
Login
To issue a token, we can use the createToken method. This token can be issued once the user authentication has succeeded. For example, when a user provides their email/username and password during login, we can issue a token immediately after the authentication process is successful.
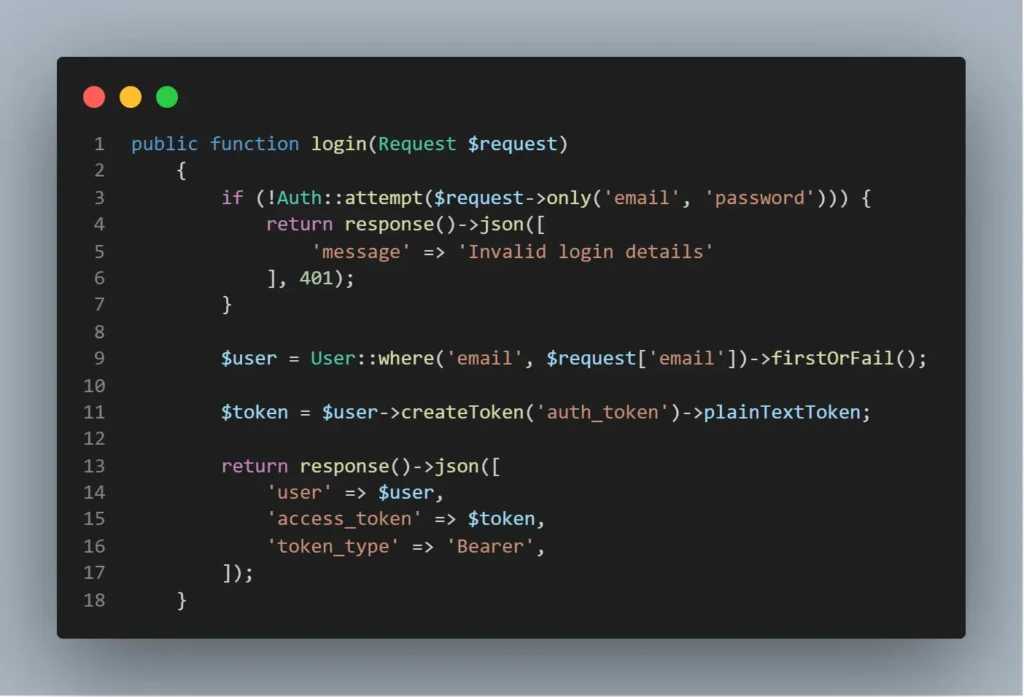
Registration
We can also issue a token immediately after a user has been registered to our system.
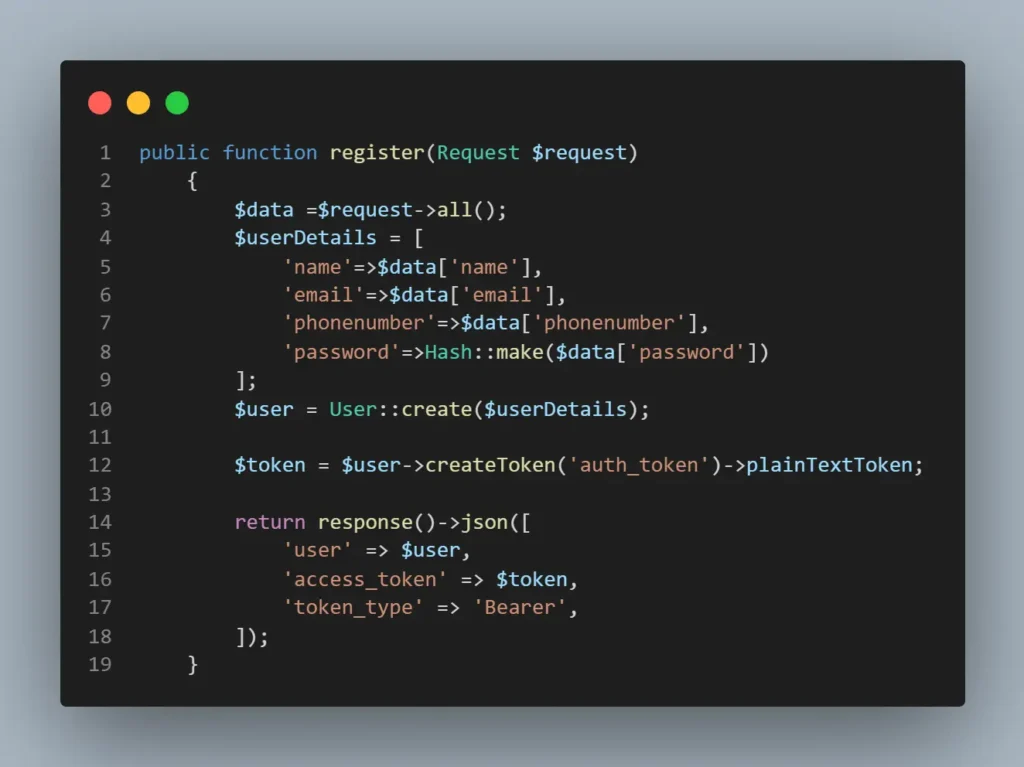
By attaching the API token to the response body, we can retrieve the API token in our Single Page Application/Mobile Application in the frontend and store it. We can then use the stored API token to make requests to the server by attaching it to the headers of all requests.
Logout
To logout a user, we can revoke an API Token and render it invalid. To do so, we have to make an API request to the logout endpoint which implements the delete() method. We have to attach the token to the header of the request in order to revoke the token and hence log out a user.
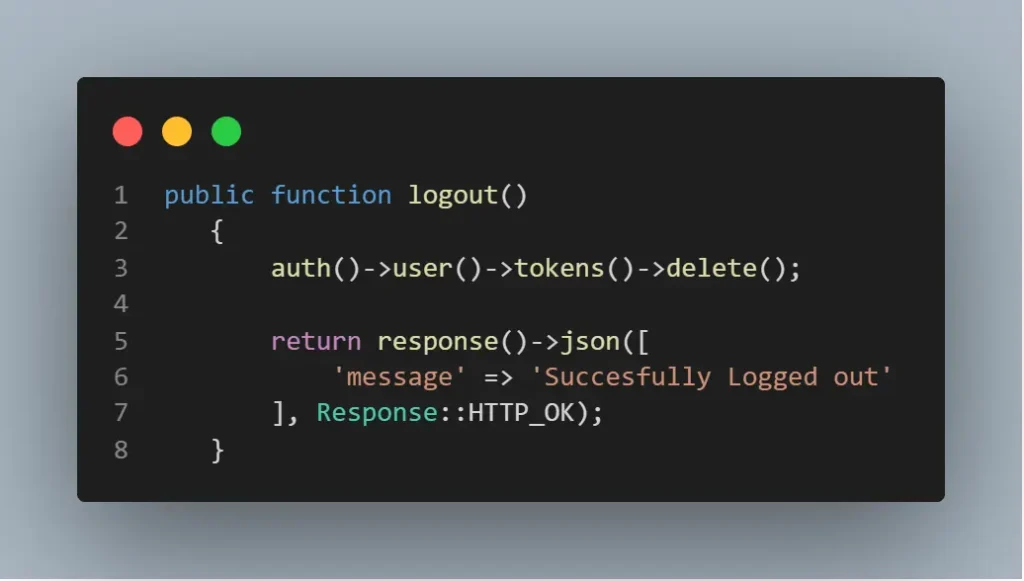
Protecting routes
The only way to protect routes so that all the incoming requests are authenticated is by attaching the sanctum authentication guard to our API routes within the routes/api.php file. This can be done by adding an auth:sanctum middleware to the routes that require protection. In our example, the logout route requires the middleware because we want it to be only accessible if a user is signed in.
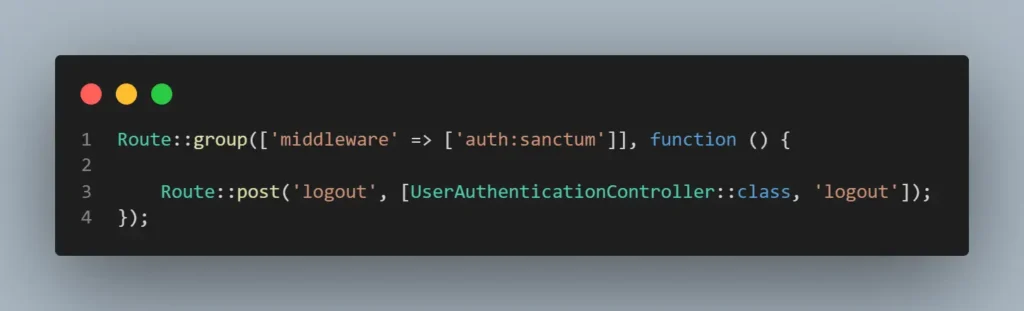
In this example, I have created a route group that will contain all the routes that need the auth:sanctum middleware
Laravel Sanctum vs Laravel Passport
Both Laravel Sanctum and Laravel Passport provide ways of API authentication. The main difference between the two is that Laravel Sanctum does not use OAuth Protocol while Laravel Passport uses the OAuth protocol to authenticate and authorize requests. Also, Sanctum is easier to use and is lightweight. If you are creating a small application that is a Single Page Application or a Mobile Application you can use Laravel Sanctum for authentication. If the application you are building is massive, serves multiple clients and other third-party sites are to use the application for authentication purposes, you can use Passport.
Conclusion
Laravel Sanctum provides an easy and simple way of implementing API authentication for your frontend application. It is lightweight and thus easy to use and ship to production. This is because it does not use encryption keys to authenticate and thus has less infrastructure when shipping to production. I hope this article was able to show you how to implement simple authentication using Laravel Sanctum. If you have any questions, feel free to ask them in the comment section and thank you for reading.